Google Drive API with Python
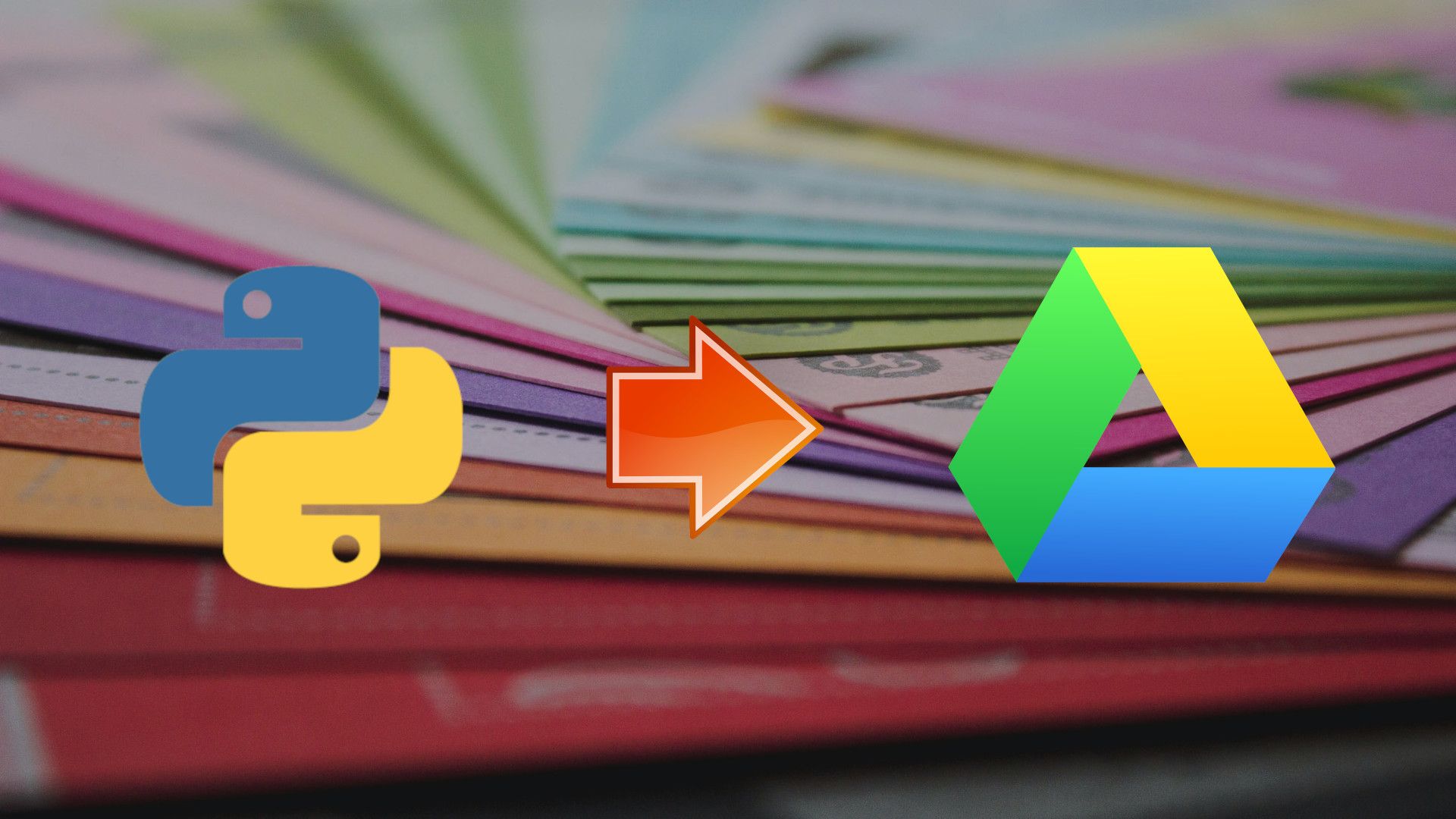
This is a follow-up to a previous post on how to connect to Google Drive using a service account with Python, for this post we are going to see how to do some common operations like downloading and uploading files, searching, copying, and deleting.
Most of the operations shown in this post are using the methods from the Files resource. Once we have built a service object, we can make calls to the Files resource by calling functions on service.files()
.
Depending on the operation we want to perform, we will need to use the appropriate scope, for example, to download a file it will be enough to use https://www.googleapis.com/auth/drive.readonly
, but for these code snippets, I will be using https://www.googleapis.com/auth/drive
to have full access, available scopes can be found here. Now let’s see some of the operations:
Searching files
To search files we make use of the list function, there is a parameter called “q” for filtering and searching, to search a file by name
:
results = service.files()
.list(q=f"name = '{file_name}'",
pageSize=10, fields="nextPageToken, files(id, name)").execute()
items = results.get('files', [])
The “q” parameter supports combining different criteria, for example, to search by mimeType
and name
:
results = service.files()
.list(q=f"mimeType='application/vnd.google-apps.folder' and name = '{folder}'",
pageSize=10, fields="nextPageToken, files(id, name)").execute()
items = results.get('files', [])
The above code search for a folder with a given name, more examples can be found here.
Downloading files
To download files we make use of the get_media function, we pass the file id as a parameter:
request = service.files().get_media(fileId=file_id)
fh = io.FileIO(file_path, 'w')
downloader = MediaIoBaseDownload(fh, request)
done = False
while done is False:
status, done = downloader.next_chunk()
print("Download %d%%." % int(status.progress() * 100))
MediaIoBaseDownload
from the googleapiclient.http
package is used to download media resources. To download Google Workspace documents such as Sheets and Slides, use the export_media
function instead.
Uploading files
To upload a file MediaFileUpload
from the googleapiclient.http
package is used, we need to pass the path to the file we want to upload and the mime type of the file, for that we can use the mimetypes
library to try to guess the type, the parent folder can also be specified in the metadata file.
file_path = os.path.join(folder, file_name)
mime_type = mimetypes.guess_type(file_path)
file_metadata = {'name': file_name}
if parent_folder_id:
file_metadata['parents'] = [parent_folder_id]
media = MediaFileUpload(file_path, mimetype=mime_type[0])
file = service.files().create(body=file_metadata,
media_body=media,
fields='id').execute()
Deleting files
It is simple to delete a file, we just need to pass the file id to the delete
method, which will delete the file permanently.
service.files().delete(fileId=file_id).execute()
Copying files
Similar to the delete operation, all we need to pass to copy a file is the file id to create a new copy in the same location as the original file, but we can specify a new name and/or a new parent folder in the file’s metadata.
file_metadata = {}
if parent_folder_id:
file_metadata['parents'] = [parent_folder_id]
if new_name:
file_metadata['name'] = new_name
file = service.files().copy(fileId=file_id,
body=file_metadata,
fields='id').execute()
I hope these small examples can serve as a base to figure out how to make more operations with the Google Drive API, the complete code can be found here.